Create Event Calendar with jQuery, PHP and MySQL
Event Calendar is an important feature of web applications to allow users to add and view events in a Calendar. In this tutorial you will learn how to create event calendar with jQuery, PHP and MySQL. We will use Bootstrap Calendar plugin to create event calendar and load events dynamically from MySQL database by making Ajax request to server side script. The tutorial explained in easy steps with live demo of event calendar and link to download source code of live demo.
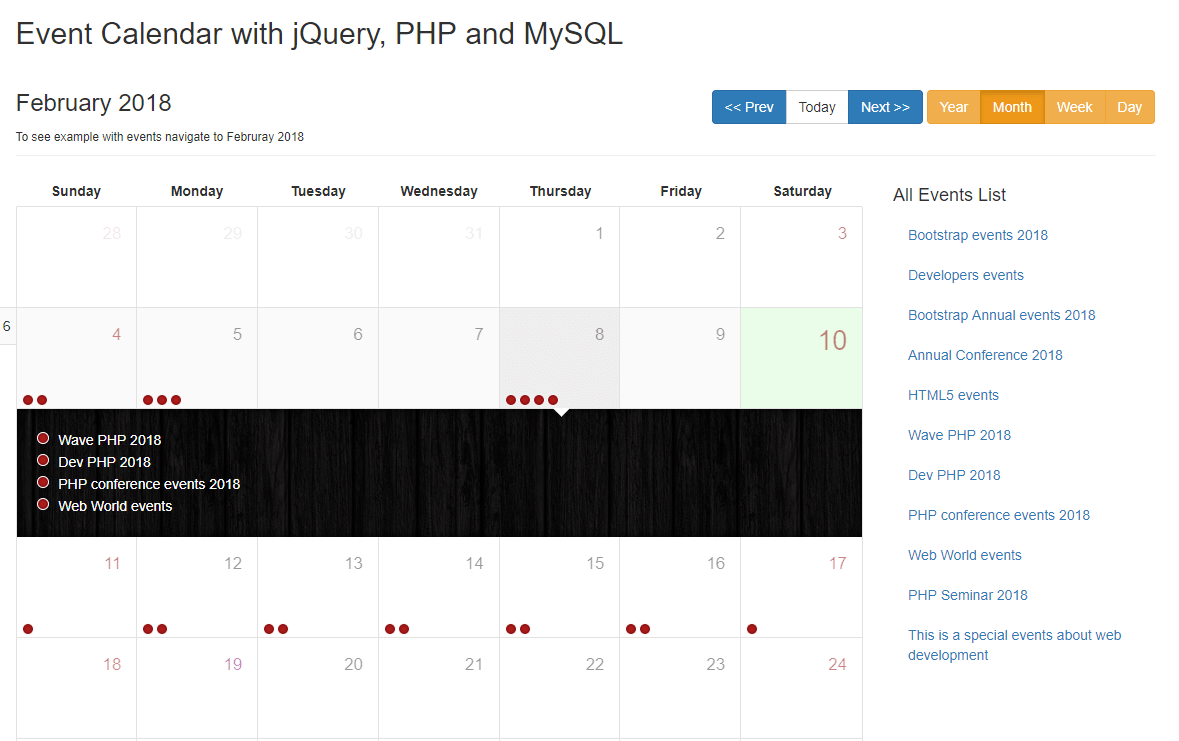
So let’s start coding. As we will cover this tutorial with live demo to create event calendar with jQuery, PHP & MySQL, so the file structure for this example is following.
Step1: Create MySQL Database Table
As we will create dynamic event calendar, so first we will create MySQL database table events by using below query.
we will also insert few event records in this table using below queries.
Step2: Include Bootstrap and jQuery Files
As we will cover this tutorial with Bootstrap calendar plugin, so we will need to include Bootstrap, jQuery and Bootstrap Calendar plugin files. We will include below files at top within head tag.
We also need to inlcude below files at the bottom of page before closing body tag.
Step3: Design Event Calendar HTML
In index.php file, we will create event calendar HTML according to Bootstrap Calendar plugin with calendar buttons.
Step4: Create Event Calendar with Bootstrap Calendar Plugin
In events.js file, we will implement Bootstrap Calendar plugin to create event calendar. We will make Ajax request to load events dynamically from MySQL database by calling event.php server side script on events_source option. We will also handle other configuration and functionality here related to event calendar.
Step5: Load Events Data from MySQL Database
Now finally in event.php, we will get events records from MySQL database and return data in JSON format according to required data format for Bootstrap Calendar plugin to load data in event calendar.
You can view the live demo from the Demo link and can download the script from the Download link below.
Demo [sociallocker]Download[/sociallocker]
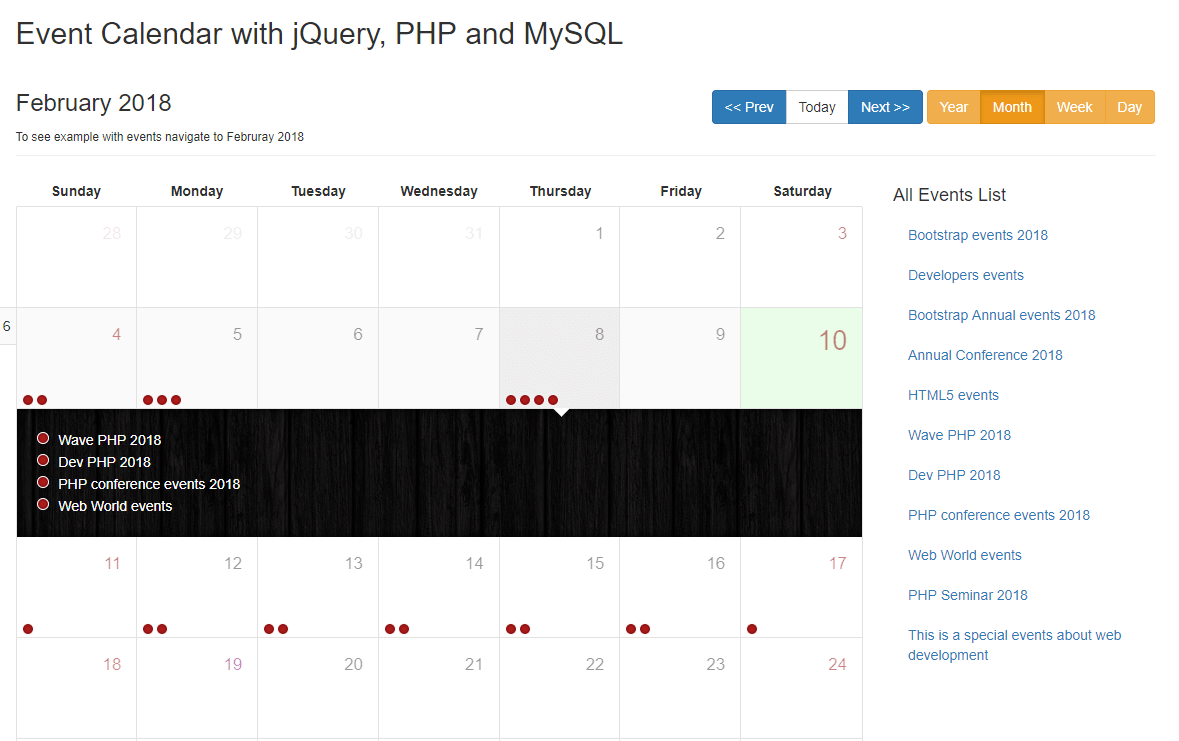
So let’s start coding. As we will cover this tutorial with live demo to create event calendar with jQuery, PHP & MySQL, so the file structure for this example is following.
- index.php
- events.js
- event.php
Step1: Create MySQL Database Table
As we will create dynamic event calendar, so first we will create MySQL database table events by using below query.
CREATE TABLE `events` (
`id` int(11) NOT NULL,
`title` varchar(255) COLLATE utf8_unicode_ci NOT NULL,
`description` varchar(255) COLLATE utf8_unicode_ci NOT NULL,
`start_date` varchar(50) COLLATE utf8_unicode_ci NOT NULL,
`end_date` varchar(50) COLLATE utf8_unicode_ci NOT NULL,
`created` datetime NOT NULL,
`status` tinyint(1) NOT NULL DEFAULT '1' COMMENT '1=Active, 0=Block'
) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci;
we will also insert few event records in this table using below queries.
INSERT INTO `events` (`id`, `title`, `description`, `start_date`, `end_date`, `created`, `status`) VALUES
(1, 'This is a special events about web development', '', '2018-02-12 00:00:00', '2018-02-16 00:00:00', '2018-02-10 00:00:00', 1),
(2, 'PHP Seminar 2018', '', '2018-02-11 00:00:00', '2018-02-17 00:00:00', '2018-02-10 00:00:00', 1),
(3, 'Bootstrap events 2018', '', '2018-02-4 00:00:00', '2018-02-4 00:00:00', '2018-02-01 00:00:00', 1),
(4, 'Developers events', '', '2018-02-04 00:00:00', '2018-02-04 00:00:00', '2018-02-01 00:00:00', 1),
(5, 'Annual Conference 2018', '', '2018-02-05 00:00:00', '2018-02-05 00:00:00', '2018-02-01 00:00:00', 1),
(6, 'Bootstrap Annual events 2018', '', '2018-02-05 00:00:00', '2018-02-05 00:00:00', '2018-02-01 00:00:00', 1),
(7, 'HTML5 events', '', '2018-02-05 00:00:00', '2018-02-05 00:00:00', '2018-02-01 00:00:00', 1),
(8, 'PHP conference events 2018', '', '2018-02-08 00:00:00', '2018-02-08 00:00:00', '2018-02-02 00:00:00', 1),
(9, 'Web World events', '', '2018-02-08 00:00:00', '2018-02-08 00:00:00', '2018-02-01 00:00:00', 1),
(10, 'Wave PHP 2018', '', '2018-02-08 00:00:00', '2018-02-08 00:00:00', '2018-02-02 00:00:00', 1),
(11, 'Dev PHP 2018', '', '2018-02-08 00:00:00', '2018-02-08 00:00:00', '2018-02-01 00:00:00', 1);
Step2: Include Bootstrap and jQuery Files
As we will cover this tutorial with Bootstrap calendar plugin, so we will need to include Bootstrap, jQuery and Bootstrap Calendar plugin files. We will include below files at top within head tag.
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/js/bootstrap.min.js"></script>
<link rel="stylesheet" href="css/calendar.css">
We also need to inlcude below files at the bottom of page before closing body tag.
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/underscore.js/1.8.3/underscore-min.js"></script>
<script type="text/javascript" src="js/calendar.js"></script>
<script type="text/javascript" src="js/events.js"></script>
Step3: Design Event Calendar HTML
In index.php file, we will create event calendar HTML according to Bootstrap Calendar plugin with calendar buttons.
<div class="container">
<div class="page-header">
<div class="pull-right form-inline">
<div class="btn-group">
<button class="btn btn-primary" data-calendar-nav="prev"><< Prev</button>
<button class="btn btn-default" data-calendar-nav="today">Today</button>
<button class="btn btn-primary" data-calendar-nav="next">Next >></button>
</div>
<div class="btn-group">
<button class="btn btn-warning" data-calendar-view="year">Year</button>
<button class="btn btn-warning active" data-calendar-view="month">Month</button>
<button class="btn btn-warning" data-calendar-view="week">Week</button>
<button class="btn btn-warning" data-calendar-view="day">Day</button>
</div>
</div>
<h3></h3>
<small>To see example with events navigate to Februray 2018</small>
</div>
<div class="row">
<div class="col-md-9">
<div id="showEventCalendar"></div>
</div>
<div class="col-md-3">
<h4>All Events List</h4>
<ul id="eventlist" class="nav nav-list"></ul>
</div>
</div>
</div>
Step4: Create Event Calendar with Bootstrap Calendar Plugin
In events.js file, we will implement Bootstrap Calendar plugin to create event calendar. We will make Ajax request to load events dynamically from MySQL database by calling event.php server side script on events_source option. We will also handle other configuration and functionality here related to event calendar.
(function($) {
"use strict";
var options = {
events_source: 'event.php',
view: 'month',
tmpl_path: 'tmpls/',
tmpl_cache: false,
day: '2018-02-28',
onAfterEventsLoad: function(events) {
if(!events) {
return;
}
var list = $('#eventlist');
list.html('');
$.each(events, function(key, val) {
$(document.createElement('li'))
.html('' + val.title + '')
.appendTo(list);
});
},
onAfterViewLoad: function(view) {
$('.page-header h3').text(this.getTitle());
$('.btn-group button').removeClass('active');
$('button[data-calendar-view="' + view + '"]').addClass('active');
},
classes: {
months: {
general: 'label'
}
}
};
var calendar = $('#showEventCalendar').calendar(options);
$('.btn-group button[data-calendar-nav]').each(function() {
var $this = $(this);
$this.click(function() {
calendar.navigate($this.data('calendar-nav'));
});
});
$('.btn-group button[data-calendar-view]').each(function() {
var $this = $(this);
$this.click(function() {
calendar.view($this.data('calendar-view'));
});
});
$('#first_day').change(function(){
var value = $(this).val();
value = value.length ? parseInt(value) : null;
calendar.setOptions({first_day: value});
calendar.view();
});
$('#language').change(function(){
calendar.setLanguage($(this).val());
calendar.view();
});
$('#events-in-modal').change(function(){
var val = $(this).is(':checked') ? $(this).val() : null;
calendar.setOptions({modal: val});
});
$('#format-12-hours').change(function(){
var val = $(this).is(':checked') ? true : false;
calendar.setOptions({format12: val});
calendar.view();
});
$('#show_wbn').change(function(){
var val = $(this).is(':checked') ? true : false;
calendar.setOptions({display_week_numbers: val});
calendar.view();
});
$('#show_wb').change(function(){
var val = $(this).is(':checked') ? true : false;
calendar.setOptions({weekbox: val});
calendar.view();
});
}(jQuery));
Step5: Load Events Data from MySQL Database
Now finally in event.php, we will get events records from MySQL database and return data in JSON format according to required data format for Bootstrap Calendar plugin to load data in event calendar.
<?php
include_once("db_connect.php");
$sqlEvents = "SELECT id, title, start_date, end_date FROM events LIMIT 20";
$resultset = mysqli_query($conn, $sqlEvents) or die("database error:". mysqli_error($conn));
$calendar = array();
while( $rows = mysqli_fetch_assoc($resultset) ) {
// convert date to milliseconds
$start = strtotime($rows['start_date']) * 1000;
$end = strtotime($rows['end_date']) * 1000;
$calendar[] = array(
'id' =>$rows['id'],
'title' => $rows['title'],
'url' => "#",
"class" => 'event-important',
'start' => "$start",
'end' => "$end"
);
}
$calendarData = array(
"success" => 1,
"result"=>$calendar);
echo json_encode($calendarData);
?>
You can view the live demo from the Demo link and can download the script from the Download link below.
Demo [sociallocker]Download[/sociallocker]
Hi! I integrate this code for my system and I use mssql server so I can get values from db but these events cannot view on the calendar can you help me?
BalasHapusYou have awesome tutorials. Thank you for this code. Saved me a lot of work.
BalasHapusThanks for the post.
BalasHapusHow to refresh the calendar with new data if I will add new event or edit.
I set a new event in calendar for other months but it does not show for other months. it only shows events set for February . Any help?
BalasHapusthanks very nice
BalasHapusI have just added current month event and its displaying when click Todays to show current month events. Please everything again!
BalasHapusIts will be refreshed automatically when page reloaded after adding new events or edit it. Thanks!
BalasHapusYou're welcome! Thanks!
BalasHapusIt should display when view next previous or today's events from calendar. Thanks!
BalasHapusHello It's working for me now.
BalasHapusThanks
how do i chance the month February to September please teach me
BalasHapusThank you so much for this Event Calendar. Just one question how do I set the default month on page load to the current month?
BalasHapusMany thanks
how to add form for adding new event or edit it thanks before
BalasHapusdaywise event is not showing properly on given time. Please check and let me update. it's urgent for me.
BalasHapusHow can I change the february to october?
BalasHapusDay wise and week wise event sorting is not working.
BalasHapusevent is not showing according to time in daywise tab and week is showing 20 days old.
please check and your earlier response is highly appreciable.
I have already
Where are css files.
BalasHapus